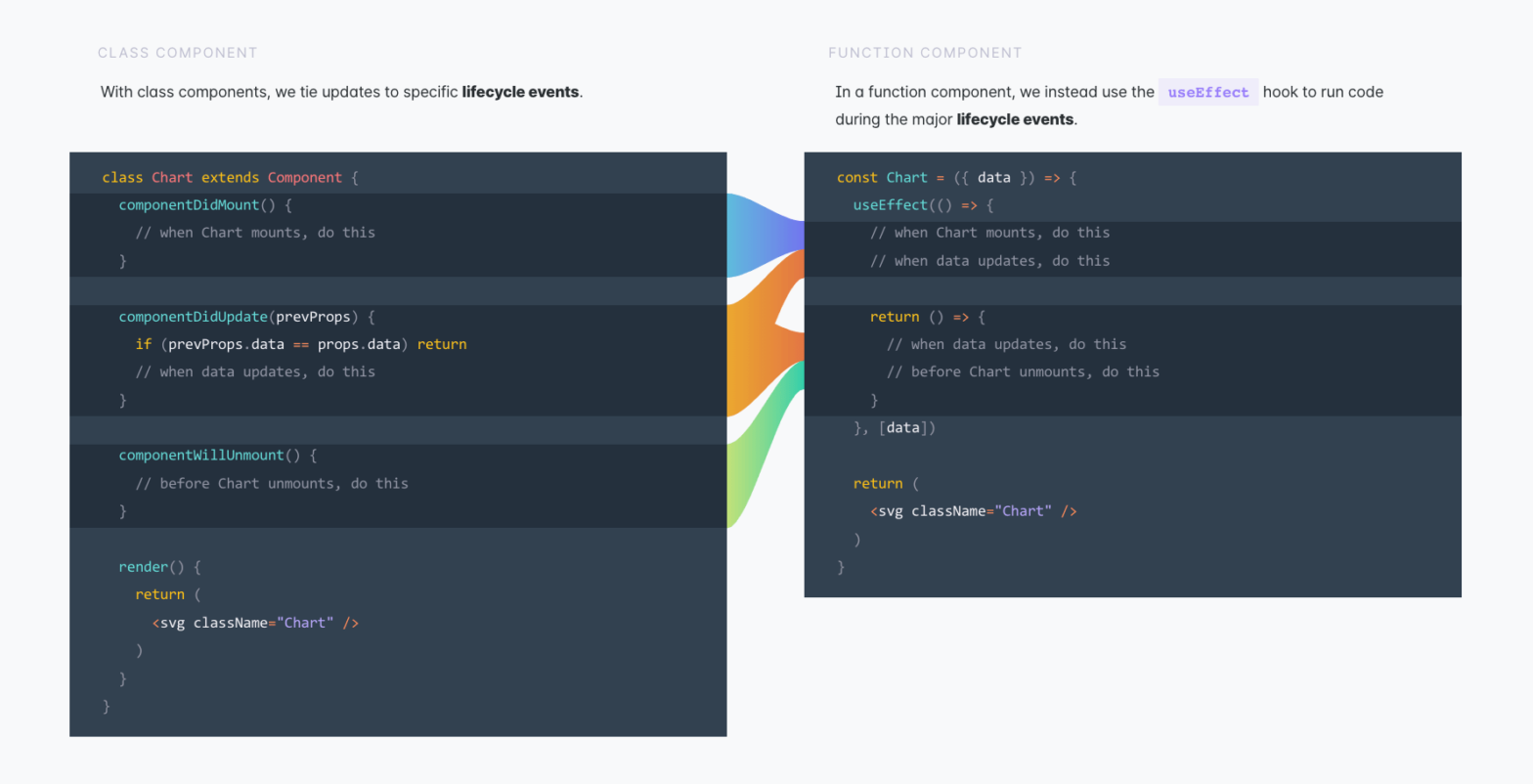
React introduced hooks one year ago, and they’ve been a game-changer for a lot of developers. There are tons of how-to introduction resources out there, but I want to talk about the fundamental mindset change when switching from React class components to function components + hooks. The code-flow visualisations between the class component and function …