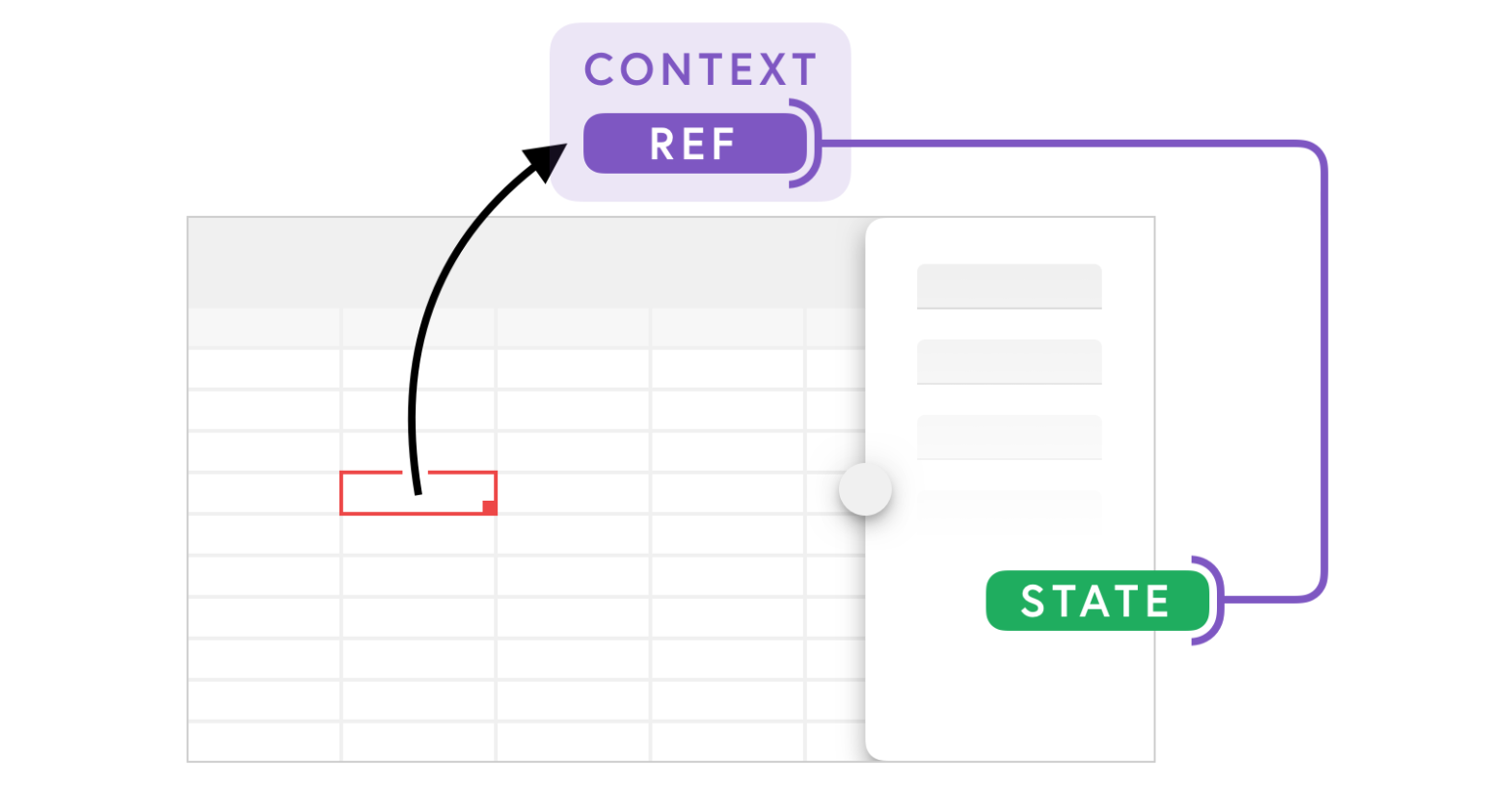
Sidney Alcantara who works on Firetable, a product which features a data grid and an adjacent side drawer. When clicking a cell in the table, the side drawer opens with info about the current cell. Initially they lifted up the state, but that caused performance issues: The problem was whenever the user selected a cell …
Continue reading “How to useRef
to Fix React Performance Issues”