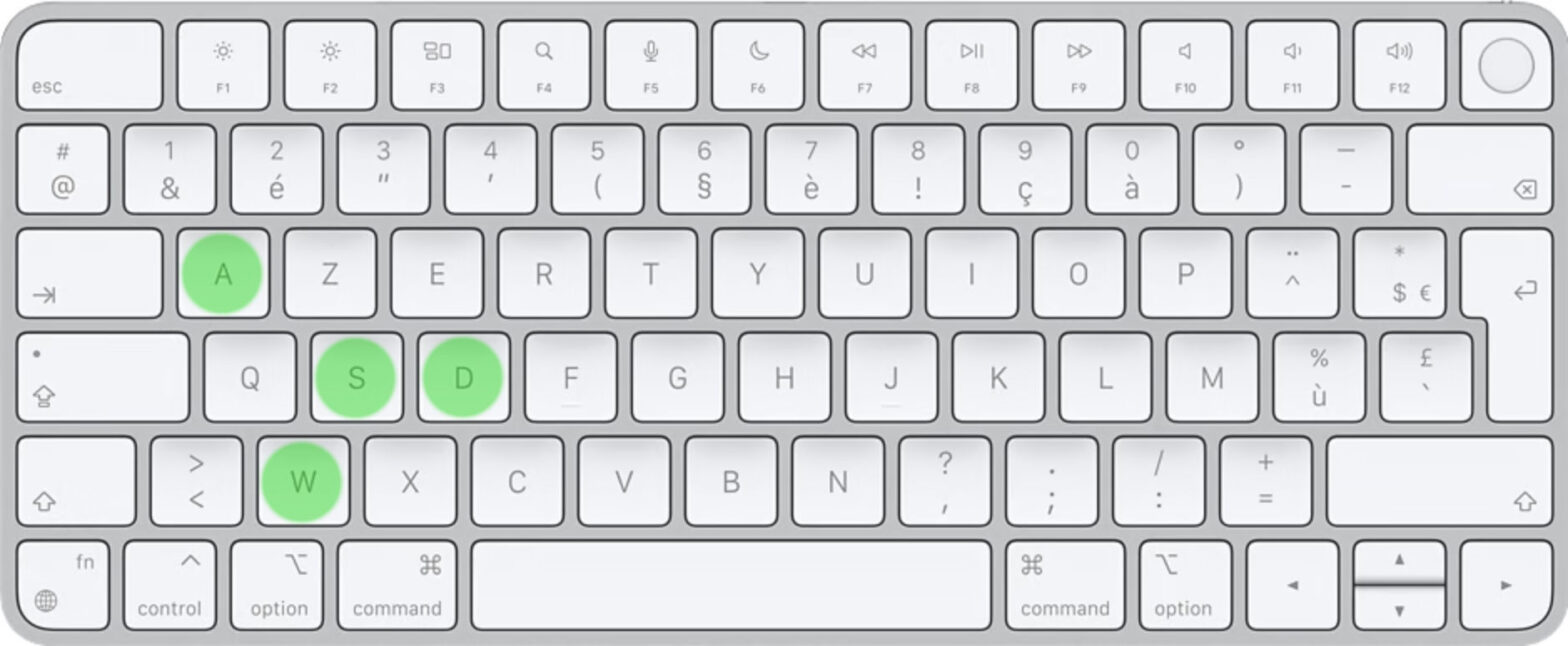
Beware when evaluating KeyboardEvent.key, as it doesn’t play nice with non-QWERTY keyboard layouts.
A rather geeky/technical weblog, est. 2001, by Bramus
WASD
Controls on the Web: Don’t use KeyboardEvent.key
but use KeyboardEvent.code
Handy helper tool by Wes Bos: simply press any key and see the results for KeyboardEvent.which, KeyboardEvent.key,KeyboardEvent.code, etc. As a user with an AZERTY keyboard layout I often have a broken experience on sites that respond to first row of keys, e.g. QWERTY. As those sites respond to KeyboardEvent.key — which differs from layout to …
Continue reading “Easily see the JavaScript Keyboard Event KeyCodes with keycode.info”
AbortController
One of the new features that was added in Chrome 88 is the ability to pass an AbortController‘s signal into addEventListener. const controller = new AbortController(); const { signal } = controller; document.querySelector(‘…’).addEventListener(‘foo’, (e) => { // … }, {signal}); By calling controller.abort();, you can remove the listener from the event target, just like when …
Continue reading “Cancel JavaScript Event Listeners with AbortController
“
Eric Bidelman has bundled lots of code snippets around change events that can get triggered in the browser: Changes range from simple things like DOM mutations and catching client-side errors to more complex notifications like knowing when the user’s battery is about to run out. The thing that remains constant are the ways to deal …
A jQuery/Zepto plugin I’ve been using a lot lately to debounce (= attach with delay) event handlers. To be applied on scroll events for example, as you don’t want one long single scroll to pull down the performance of your webpage by constantly triggering the attached handling function. $(window).on(‘scroll’, function(e) { // update stuff *after* …
Événement is a very simple event dispatching library for PHP. It has the same design goals as Silex and Pimple, to empower the user while staying concise and simple. Concise indeed. At the core it’s a tad above 50 lines of codes. Listening for events: $emitter->on(‘user.created’, function (User $user) use ($logger) { $logger->log(sprintf(“User ‘%s’ was …
var beingWatched = {}; function whatHappened(change) { console.log(change.name + ” was ” + change.type + ” and is now ” + change.object[change.name]); } function somethingChanged(changes) { changes.forEach(whatHappened); } Object.observe(beingWatched, somethingChanged); beingWatched.a = “foo”; // new beingWatched.a = “bar”; // updated beingWatched.a = “bar”; // no change beingWatched.b = “amazing”; // new Object.observe() lets you add …
Inspired upon Node.js: <?php require ‘vendor/autoload.php’; $stack = new React\Espresso\Stack(function ($request, $response) { $response->writeHead(200, array(‘Content-Type’ => ‘text/plain’)); $response->end(‘Hello World\n’); }); echo ‘Server running at http://127.0.0.1:1337’ . PHP_EOL; $stack->listen(1337); React →