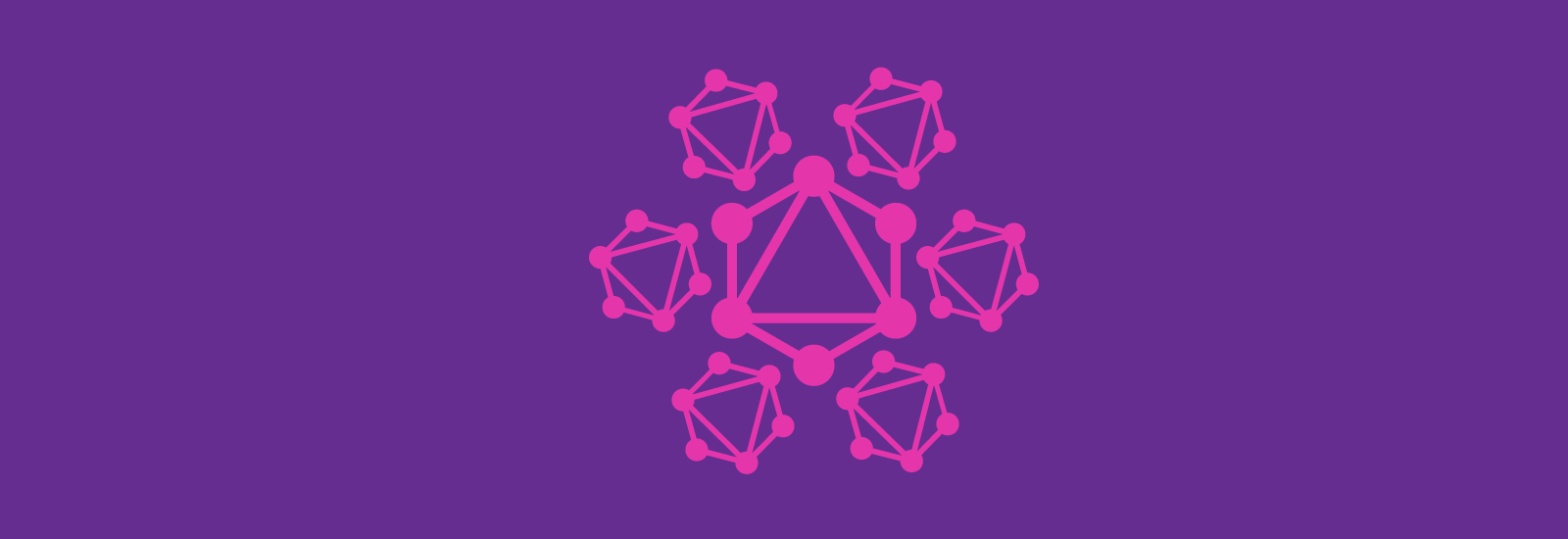
Using Apollo Client’s InMemoryCache and Local Resolvers, it’s possible to have it query your Redux store: import store from "./Store"; const typeDefs = gql` type Query { users: [User] } type User { groups: [Group] } type Group {} `; const client = new ApolloClient({ cache: new InMemoryCache(), typeDefs, resolvers: { Query: { users: () …